Types of Inheritance in Java: A Comprehensive Guide
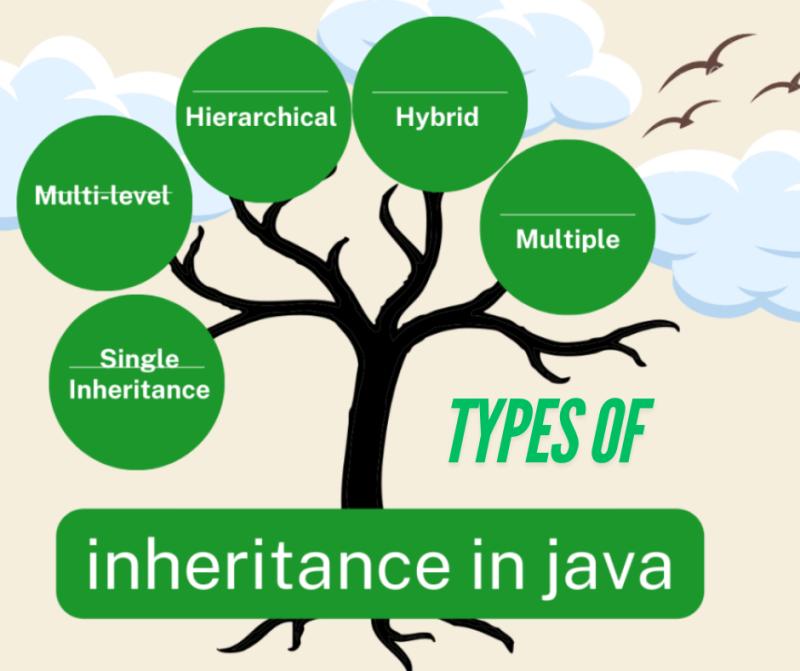
Inheritance is a core principle of OOP that allows one or more new classes to inherit data characteristics and functions from one or more existing classes. In Java, inheritance enables code reusability and brings in a hierarchical relationship between the classes which helps to keep the classes organized and maintainable as well as extensible. Inheritance allows you to create new classes based on the existing ones so that, rather than starting from zero, you can continue existing work. Now, let us discuss various types of inheritance in Java.
What is Inheritance?
In Java, inheritance enables one class (subclass or child class) to reuse fields and methods from another class (superclass or parent class). That relationship establishes one-way hierarchy to subclass by gaining all non-private members of the superclass which means that they are available for use or overridden. Please note that Java has a single inheritance only, hence a class can inherit from one and only one parent class.
Why Use Inheritance?
Inheritance helps in:
Code Reusability: You don’t need to write the same code repeatedly.
Maintainability: Changes in a superclass automatically propagate to subclasses, reducing redundant code updates.
Polymorphism: Inheritance allows the same method to behave differently in subclasses, supporting method overriding.
Hierarchy Creation: By building a class hierarchy, you can better organize your code and use abstraction.
Types of Inheritance in Java
Java supports several types of inheritance, although, by design, some are restricted due to Java’s limitations, such as the absence of direct multiple inheritance. Here are the main types:
Single Inheritance
Multilevel Inheritance
Hierarchical Inheritance
Multiple Inheritance (with Interfaces)
Hybrid Inheritance (with Interfaces)
Let’s discuss each of these in detail.
1. Single Inheritance
Single Inheritance occurs when a subclass inherits from only one superclass. This is the simplest form of inheritance and establishes a straightforward parent-child relationship between two classes.
Example Concept: Suppose you have a class Animal, and a class Dog that inherits from Animal. In this scenario, Dog acquires the properties and behaviors of Animal but can also have its own unique properties.
Advantages:
Simple structure that’s easy to understand and maintain.
Prevents ambiguity since the subclass has only one superclass.
Limitation:
In cases where functionality needs to be inherited from multiple sources, single inheritance may not be sufficient. However, Java provides interfaces as a workaround.
2. Multilevel Inheritance
Multilevel Inheritance is a type of inheritance where a class derives from another derived class, creating a chain of inheritance. Here, the subclass becomes the parent class for another class, thus forming a hierarchy.
Example Concept: Suppose we have a class Animal, and a class Mammal inherits from Animal, followed by a class Dog that inherits from Mammal. This structure creates a multilevel inheritance hierarchy (Animal → Mammal → Dog).
Advantages:
Allows for a deeper level of inheritance, enabling more specialized classes.
Promotes code reuse by passing inherited features down multiple levels.
Limitation:
Complex structures can be challenging to debug and maintain, especially as more levels are added.
3. Hierarchical Inheritance
Hierarchical Inheritance occurs when multiple subclasses inherit from a single superclass. This structure allows multiple classes to share common features of a superclass while also having their own distinct characteristics.
Example Concept: Suppose Animal is a superclass, and both Dog and Cat are subclasses of Animal. Here, Dog and Cat inherit the properties of Animal, but each subclass can have its unique attributes.
Advantages:
Useful when you want different classes to share common functionality from a single superclass.
Provides better code organization as shared methods and properties are managed in a common superclass.
Limitation:
Changes in the superclass can affect all subclasses, potentially leading to unintended consequences if subclasses heavily rely on inherited functionality.
4. Multiple Inheritance (with Interfaces)
Java does not support Multiple Inheritance with classes directly, meaning a class cannot inherit from more than one superclass. This restriction avoids ambiguity when multiple superclasses have methods with the same name and signature. However, multiple inheritance can be achieved in Java through interfaces.
Interfaces allow a class to implement multiple sets of behaviors without inheriting the actual implementation. This way, a class can implement multiple interfaces and simulate multiple inheritance.
Example Concept: Suppose you have two interfaces, Runnable and Swimmable, which both define distinct behaviors. A class Dog could implement both interfaces, thus inheriting the ability to both run and swim.
Advantages:
Eliminates ambiguity issues by separating behaviors into interfaces rather than classes.
Promotes modular design as a class can implement only the interfaces it needs.
Limitation:
While interfaces provide method signatures, they do not offer method implementations, so every implementing class must define the method behavior, potentially leading to duplicated code.
5. Hybrid Inheritance (with Interfaces)
Hybrid Inheritance is a combination of two or more types of inheritance. For example, you may have a mix of single, multilevel, and multiple inheritance in a program. Java supports hybrid inheritance through a combination of classes and interfaces, as classes alone do not support multiple inheritance.
Example Concept: Consider a class Vehicle, with two interfaces, LandVehicle and WaterVehicle. A class AmphibiousCar can extend Vehicle and implement both LandVehicle and WaterVehicle, thus combining different types of inheritance.
Advantages:
Enables a flexible design where multiple inheritance types can be used based on specific requirements.
Allows developers to make the best use of different inheritance types in one cohesive structure.
Limitation:
Can lead to complex and hard-to-read code if not implemented carefully, as combining multiple inheritance types can complicate the hierarchy.
Key Points About Inheritance in Java
Constructor Calling: When a subclass object is created, the superclass’s constructor is automatically invoked. This process initializes the superclass properties before the subclass properties are initialized.
Method Overriding: In inheritance, a subclass can redefine a method from its superclass, a concept known as overriding. This allows subclasses to provide specific implementations while still inheriting general behavior.
The super Keyword: The super keyword allows subclasses to refer to their superclass’s methods or constructors. This is useful for accessing overridden methods or specific constructor versions in the superclass.
Protected Access: Java’s protected access modifier plays an important role in inheritance, as it allows subclass access to superclass members while still keeping them hidden from non-subclass code in other packages.
Polymorphism: Polymorphism is closely linked with inheritance. It allows methods to perform differently based on the object that invokes them. This dynamic method dispatch makes inheritance even more powerful, allowing subclasses to be treated as instances of their superclass.
Summary
Inheritance is a key feature in Java that enables new classes to inherit characteristics from existing ones, leading to code reusability, better organization, and support for polymorphism. Java supports several inheritance types, including single, multilevel, and hierarchical inheritance. In a hierarchical inheritance program in Java, multiple subclasses can inherit from a single superclass. This allows multiple classes to share common behavior from a single parent class. Java also supports multiple
Comments (1)
Astro Arun Pandit
6
Good !!.. Full Concept about inheritance..