Full Stack Development: A Comprehensive Guide for Web Developers
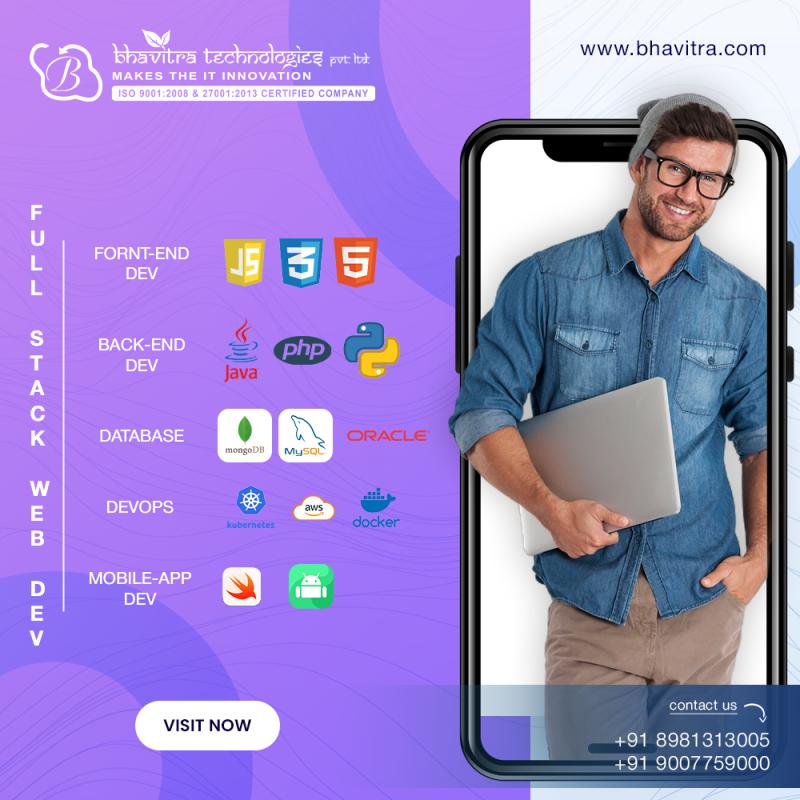
Full-stack web development is the practice of building both the frontend and backend of a web application. This guide by low-cost web development company in India will walk you through the essential technologies, concepts, and a simple project to illustrate how a full-stack application is built.
#### 1. **Understanding Full Stack Development**
Full-stack development involves working with both the **frontend** (what users see) and the **backend** (the server, database, and application logic). Here's a quick overview:
- **Frontend:** The client-side of the application, dealing with the user interface and experience. Technologies include HTML, CSS, JavaScript, and frameworks like React.js, Angular, and Vue.js.
- **Backend:** The server-side of the application, managing the database, server, and business logic. Technologies include Node.js, Express.js, Django, Ruby on Rails, and databases like MySQL and MongoDB.
#### 2. **Setting Up Your Environment**
Before diving into code, you'll need to set up a development environment. This typically includes:
- **Text Editor/IDE:** VS Code, Sublime Text, or WebStorm.
- **Node.js and npm:** Node.js is a JavaScript runtime, and npm is its package manager. Install it from [Node.js official website](https://nodejs.org/).
- **Git:** For version control. Install it from [Git official website](https://git-scm.com/).
- **Database:** For this guide, we’ll use MongoDB, which is a NoSQL database.
#### 3. **Creating a Simple Full Stack Application**
Let’s build a simple full-stack application where users can create, read, update, and delete (CRUD) notes.
##### **Step 1: Backend Setup with Node.js and Express**
1. **Initialize the Project:**
```bash
mkdir fullstack-app
cd fullstack-app
npm init -y
```
2. **Install Dependencies:**
```bash
npm install express mongoose body-parser cors
```
3. **Set Up Express Server:**
Create a file named `server.js`:
```javascript
const express = require('express');
const mongoose = require('mongoose');
const bodyParser = require('body-parser');
const cors = require('cors');
const app = express();
app.use(bodyParser.json());
app.use(cors());
mongoose.connect('mongodb://localhost:27017/fullstack-app', {
useNewUrlParser: true,
useUnifiedTopology: true,
});
const noteSchema = new mongoose.Schema({
title: String,
content: String,
});
const Note = mongoose.model('Note', noteSchema);
app.get('/notes', async (req, res) => {
const notes = await Note.find();
res.json(notes);
});
app.post('/notes', async (req, res) => {
const newNote = new Note({
title: req.body.title,
content: req.body.content,
});
await newNote.save();
res.json(newNote);
});
app.put('/notes/:id', async (req, res) => {
const updatedNote = await Note.findByIdAndUpdate(req.params.id, req.body, { new: true });
res.json(updatedNote);
});
app.delete('/notes/:id', async (req, res) => {
await Note.findByIdAndDelete(req.params.id);
res.json({ message: 'Note deleted' });
});
app.listen(3001, () => {
console.log('Server is running on port 3001');
});
```
4. **Run the Server:**
```bash
node server.js
```
##### **Step 2: Frontend Setup with React**
1. **Create a React App:**
```bash
npx create-react-app client
cd client
```
2. **Install Axios for API Calls:**
```bash
npm install axios
```
3. **Build the Frontend:**
Edit the `App.js` file:
```javascript
import React, { useState, useEffect } from 'react';
import axios from 'axios';
function App() {
const [notes, setNotes] = useState([]);
const [title, setTitle] = useState('');
const [content, setContent] = useState('');
useEffect(() => {
axios.get('http://localhost:3001/notes')
.then(response => setNotes(response.data))
.catch(error => console.error(error));
}, []);
const addNote = () => {
axios.post('http://localhost:3001/notes', { title, content })
.then(response => setNotes([...notes, response.data]))
.catch(error => console.error(error));
};
const deleteNote = (id) => {
axios.delete(`http://localhost:3001/notes/${id}`)
.then(() => setNotes(notes.filter(note => note._id !== id)))
.catch(error => console.error(error));
};
return (
<div>
<h1>Notes</h1>
<input
placeholder="Title"
value={title}
onChange={e => setTitle(e.target.value)}
/>
<textarea
placeholder="Content"
value={content}
onChange={e => setContent(e.target.value)}
/>
<button onClick={addNote}>Add Note</button>
<ul>
{notes.map(note => (
<li key={note._id}>
<h2>{note.title}</h2>
<p>{note.content}</p>
<button onClick={() => deleteNote(note._id)}>Delete</button>
</li>
))}
</ul>
</div>
);
}
export default App;
```
4. **Run the React App:**
```bash
npm start
```
Now, when you navigate to `http://localhost:3000` in your browser, you should see a simple notes application where you can add, view, and delete notes.
#### 4. **Understanding the Code**
- **Backend (Node.js + Express):**
- **Mongoose** is used to interact with MongoDB. It defines a schema (`noteSchema`) and a model (`Note`) for storing and retrieving notes.
- **Express** is used to set up a RESTful API with routes for creating, reading, updating, and deleting notes.
- **CORS** is enabled to allow communication between the frontend and backend running on different ports.
- **Frontend (React):**
- **useState** and **useEffect** hooks are used to manage state and side effects in the React component.
- **Axios** is used to make HTTP requests to the backend API.
- The application allows users to enter a note title and content, add it to the database, and delete existing notes.
#### 5. **Best Practices**
- **Use Environment Variables:** Store sensitive information like database URLs or API keys in environment variables.
- **Error Handling:** Always add proper error handling to both the backend and frontend to ensure the application is resilient.
- **Componentization in React:** Break down the UI into smaller components for better maintainability and reusability.
- **Security:** Implement security practices such as input validation, authentication, and authorization.
#### 6. **Next Steps**
This guide provides a foundational understanding of full-stack web development. To take your skills further:
- **Learn Authentication:** Implement user authentication using JWT or OAuth.
- **Explore Advanced Backend Features:** Dive into caching, rate limiting, and advanced database queries.
- **Frontend State Management:** Explore state management libraries like Redux for more complex applications.
- **Deployment:** Learn how to deploy your application on cloud platforms like AWS, Heroku, or DigitalOcean.
#### 7. **Conclusion**
Full-stack web development is a rewarding field that allows you to build complete applications from start to finish. By mastering both frontend and backend technologies, you can create dynamic, scalable, and robust web applications. This guide by Bhavitra Technologies is just the beginning—continue exploring, building projects, and learning to become a proficient full-stack developer.